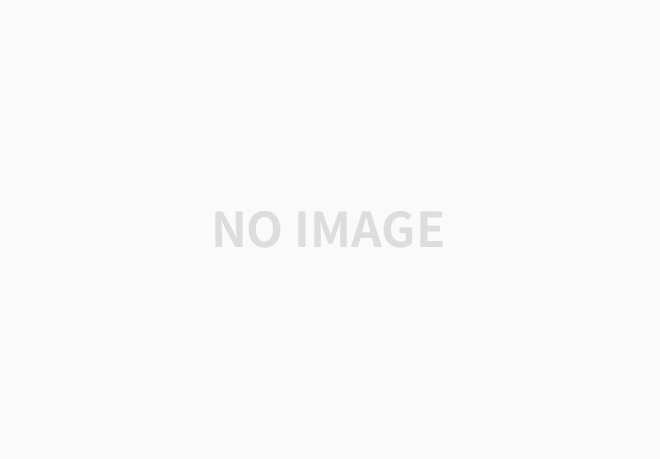
https://www.trek10.com/blog/aws-lambda-python-asyncio 에서 비교한 자료 요약.
다음 3가지 코드로 퍼포먼스 비교함.
- 병렬처리 없이 순차적으로 실행하는 코드
- aioboto3(boto3 라이브러리에 비동기 처리를 할 수 있게 해주는 라이브러리)를 이용한 병렬처리 코드
- boto3 라이브러리를 Python 기본 라이브러리인 ayncio를 이용해 작성한 병렬처리 코드
코드 구성
- 기본 순차 처리 코드
import os
import boto3
s3 = boto3.client('s3')
BUCKET_NAME = os.getenv('BUCKET_NAME')
def main():
bucket_contents = s3.list_objects_v2(Bucket=BUCKET_NAME)
objects = [
s3.get_object_acl(Bucket=BUCKET_NAME, Key=content_entry['Key'])
for content_entry in bucket_contents['Contents']
]
def handler(event, context):
return main()
- asyncio 를 이용한 boto3 코드
import asyncio
import functools
import os
import boto3
BUCKET_NAME = os.getenv('BUCKET_NAME')
s3 = boto3.client('s3')
async def main():
loop = asyncio.get_running_loop()
bucket_contents = s3.list_objects_v2(Bucket=BUCKET_NAME)
objects = await asyncio.gather(
*[
loop.run_in_executor(None, functools.partial(s3.get_object_acl, Bucket=BUCKET_NAME, Key=content_entry['Key']))
for content_entry in bucket_contents['Contents']
]
)
def handler(event, context):
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
- aioboto3와 asyncio를 이용한 코드
import asyncio
import os
import aioboto3
BUCKET_NAME = os.getenv('BUCKET_NAME')
async def main():
async with aioboto3.client('s3') as s3:
bucket_contents = await s3.list_objects_v2(Bucket=BUCKET_NAME)
objects = await asyncio.gather(
*[
s3.get_object_acl(Bucket=BUCKET_NAME, Key=content_entry['Key'])
for content_entry in bucket_contents['Contents']
]
)
def handler(event, context):
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
각 코드는 Python 3.8.x 런타임용 Lambda Function으로 구성하여 실행함.
실행 결과
결과는 다음과 같다.
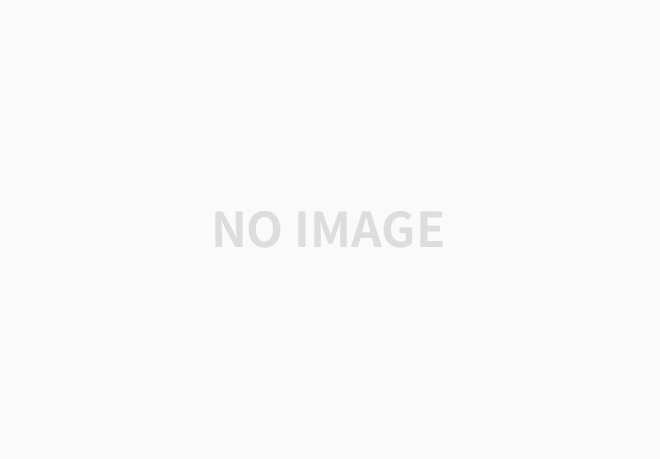
출처: https://www.trek10.com/blog/aws-lambda-python-asyncio
Memory (mb) | Sync boto3 | Async aioboto3 | Async boto3 |
128 | 4771.45 | 4792.22 | 6097.20 |
512 | 2020.62 | 1259.93 | 1446.13 |
1024 | 1888.41 | 734.59 | 707.98 |
1536 | 1921.05 | 615.03 | 486.31 |
2048 | 1824.93 | 682.80 | 483.95 |
3008 | 1799.03 | 616.14 | 572.16 |
100회 호출의 평균 실행 시간. 모든 시간은 밀리초 단위임. |
- 놀랍게도 낮은 메모리 (128mb)에서 순차 동기 호출은 비동기 방법보다 빠르다.
- 더 높은 Lambda 메모리에서 aioboto3는 boto3보다 이점이 없음.
글쓴이의 결론
- 동기식 호출(Synchronous calls)은 때로는 병렬처리만큼 빠르다.
- boto3는 기본 병렬처리만으로도 충분하고, 일부의 경우에는 aioboto3 의 퍼포먼스를 상회한다.
'AWS > Lambda' 카테고리의 다른 글
Lambda Function에서 Python용 Handler를 호출해주는 코드 (1) | 2024.11.15 |
---|---|
AWS Lambda 런타임 중 Python에서 사용가능한 기본 Package 목록 (0) | 2023.06.08 |
AWS SAM Local Testing 시 Docker desktop이 아닌 'minikube' 사용하기 (macOS 기준) (0) | 2023.02.01 |
AWS Lambda behind the scenes (0) | 2022.07.14 |
Lambda함수 내에서 사용할 수 있는 최대 CPU 개수는?? (0) | 2022.07.14 |